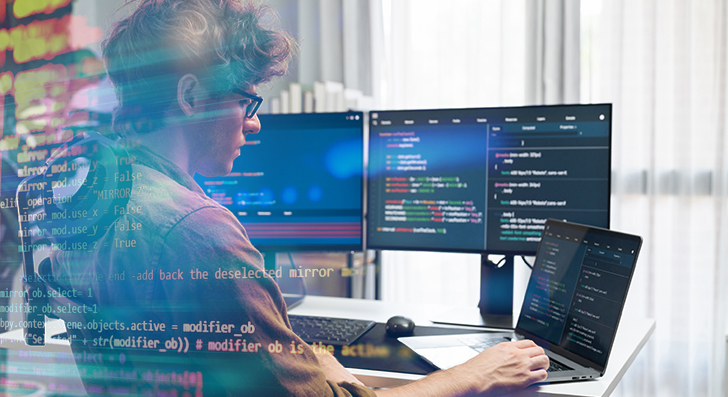
Scalability implies your application can manage growth—extra people, a lot more information, and more targeted visitors—devoid of breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to help you start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it should be aspect of one's approach from the start. Numerous apps fail if they develop rapid simply because the first style can’t cope with the extra load. To be a developer, you should Imagine early about how your technique will behave stressed.
Begin by coming up with your architecture to become adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. As a substitute, use modular design or microservices. These patterns split your application into smaller, impartial pieces. Every module or provider can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day just one. Will it need to handle a million end users or merely 100? Pick the right kind—relational or NoSQL—dependant on how your data will develop. Strategy for sharding, indexing, and backups early, Even though you don’t need to have them nevertheless.
A different vital point is to avoid hardcoding assumptions. Don’t publish code that only performs underneath latest ailments. Give thought to what would materialize if your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that assist scaling, like concept queues or function-driven methods. These assist your app manage a lot more requests without having obtaining overloaded.
After you Establish with scalability in your mind, you're not just getting ready for success—you might be decreasing future problems. A perfectly-prepared program is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the proper Databases
Picking out the proper database is usually a critical Section of developing scalable purposes. Not all databases are designed the identical, and using the wrong you can slow you down or even bring about failures as your app grows.
Get started by knowledge your info. Can it be really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a great suit. These are solid with relationships, transactions, and regularity. They also support scaling tactics like study replicas, indexing, and partitioning to manage much more website traffic and information.
In the event your info is a lot more flexible—like consumer activity logs, product or service catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured facts and can scale horizontally far more conveniently.
Also, contemplate your study and publish styles. Are you currently undertaking many reads with fewer writes? Use caching and browse replicas. Will you be handling a large publish load? Explore databases which will tackle higher publish throughput, or maybe party-primarily based info storage programs like Apache Kafka (for non permanent data streams).
It’s also intelligent to Imagine in advance. You may not require Superior scaling characteristics now, but picking a databases that supports them usually means you received’t need to switch later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your facts based upon your obtain styles. And normally monitor databases performance as you expand.
In a nutshell, the correct database is determined by your app’s structure, speed needs, And exactly how you hope it to mature. Acquire time to choose sensibly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Prevent repeating logic and remove something unnecessary. Don’t select the most sophisticated solution if a straightforward a single works. Keep the functions short, centered, and simple to test. Use profiling instruments to discover bottlenecks—places the place your code will take much too prolonged to run or works by using a lot of memory.
Future, have a look at your database queries. These normally slow matters down over the code alone. Ensure Each individual query only asks for the info you actually need to have. Steer clear of Pick out *, which fetches every thing, and instead pick unique fields. Use indexes to speed up lookups. And prevent doing too many joins, Primarily across massive tables.
For those who discover the exact same data staying asked for repeatedly, use caching. Keep the effects temporarily employing instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations once you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app additional economical.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred documents might crash whenever they have to manage one million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These steps help your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more site visitors. If every little thing goes by means of a single server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching come in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. In lieu of a person server executing every one of the operate, the load balancer routes consumers to diverse servers depending on availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it could be reused swiftly. When users ask for exactly the same information yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database when. It is possible to serve it with the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) shops details in memory for quickly obtain.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near to the person.
Caching decreases databases load, increases speed, and can make your application a lot more economical.
Use caching for things that don’t transform often. And constantly make sure your cache is up to date when facts does alter.
Briefly, load balancing and caching are simple but effective tools. Collectively, they assist your app manage additional users, remain rapidly, and Get better from issues. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you will need applications that let your app expand simply. That’s where by cloud platforms and website containers come in. They give you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t should invest in components or guess upcoming capacity. When traffic increases, you are able to include much more sources with only a few clicks or immediately utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You are able to focus on building your application in place of taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it simple to maneuver your application in between environments, from your notebook for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If just one portion of your app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual parts of your application into solutions. You could update or scale areas independently, which is perfect for overall performance and trustworthiness.
In brief, working with cloud and container resources suggests you'll be able to scale speedy, deploy very easily, and Get better speedily when problems come about. If you want your application to mature without having restrictions, begin working with these tools early. They preserve time, cut down danger, and allow you to continue to be focused on constructing, not correcting.
Monitor Almost everything
For those who don’t keep track of your software, you received’t know when issues go Mistaken. Checking allows you see how your app is doing, location challenges early, and make much better choices as your application grows. It’s a critical part of developing scalable techniques.
Start out by monitoring essential metrics like CPU use, memory, disk space, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it's going to take for buyers to load internet pages, how frequently faults materialize, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for important problems. For example, if your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This assists you fix issues speedy, generally in advance of end users even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new element and see a spike in problems or slowdowns, you are able to roll it again in advance of it triggers real destruction.
As your app grows, traffic and details enhance. With out checking, you’ll overlook indications of difficulties till it’s much too late. But with the best tools set up, you stay on top of things.
In brief, checking assists you keep the app trusted and scalable. It’s not just about recognizing failures—it’s about comprehension your system and making certain it works properly, even stressed.
Last Views
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By planning carefully, optimizing properly, and utilizing the correct equipment, you can Construct applications that grow easily without the need of breaking under pressure. Start off compact, Consider significant, and Develop sensible.